Overview :
In the world of MVC web development , you might have built various rich UI web application by using HTML user interface components say textbox, checkbox,dropdown etc.
Most of the time while designing your UI, you might have used normal HTML controls or built-in MVC helper methods.. isn't it?. but there may be a situation where MVC built-in helper methods or conventional HTML controls will not full fill your requirements. i.e., at times you may want to load dropdown items based on some condition or logic.
So to overcome this situation, we will be using custom helper methods using which we can formulate the data by adding business logic of our own.
Step 1 : Create a MVC app using VS tool.
Step 2 : Add Controller , name it as 'Home'. And create action method , name it as 'Index'.
Step 3 : Create view for Index action method.
I have added following code inside the view.
As you can observe above lines of code., I have created simple student form where I have added a custom dropdown control
"@Html.CustomGenderDropDownList("studentGender","--Select--")".
MVC does not have this type of helper method in its assembly. It is an extension method.
Now run the application and check the result.
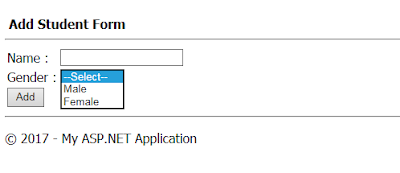
As you can see above picture, I am able to load gender dropdown items using custom dropdown helper method.
Follow below steps to implement custom dropdown helper method.
Step 1 : Create a class and name it as you like.
Step 2 : Add below line of code inside it.
I have written a method called 'CustomGenderDropDownList' which is expecting multiple parameters such as name, optional label and html attributes.
Here ,
1. 'name' parameter indicates control's name
2. 'Optional Label' indicates whether or not you want to display '--select--' as first item in your dropdown control.
I added sample list of items and added them into IEnumerable of SelectListItem object
and pass them to 'helper.DropdownList' method and return it as . MvcHtmlString object.
Note: You can pull object list from DB and add them to 'IEnumerable of SelectListItem' object
That's it. You are done.
Note :
1. If you would like to add strongly typed dropdown control , write a helper method as below
public static MvcHtmlString CustomGenderDropDownListFor<TModel, TValue>(this HtmlHelper<TModel> helper, Expression<Func<TModel, TValue>> expression, string optionalLabel, object htmlAttributes = null)
{
--Add your logic--
}
Please feel free to ask your querries. I will be glad to help you all. And Hope this article will help you guys.
User reads below articles also:
In the world of MVC web development , you might have built various rich UI web application by using HTML user interface components say textbox, checkbox,dropdown etc.
Most of the time while designing your UI, you might have used normal HTML controls or built-in MVC helper methods.. isn't it?. but there may be a situation where MVC built-in helper methods or conventional HTML controls will not full fill your requirements. i.e., at times you may want to load dropdown items based on some condition or logic.
So to overcome this situation, we will be using custom helper methods using which we can formulate the data by adding business logic of our own.
Well, today I am going to explain how to create custom dropdown helper method in MVC.
Step 1 : Create a MVC app using VS tool.
Step 2 : Add Controller , name it as 'Home'. And create action method , name it as 'Index'.
Step 3 : Create view for Index action method.
I have added following code inside the view.
@using CustomHelperMethod
<br />
<span style="font-weight:bold">Add Student Form</span>
<hr />
<table>
<tr>
<td>
Name :
</td>
<td>
@Html.TextBox("studentName")
</td>
</tr>
<tr>
<td>
Gender :
</td>
<td>
@Html.CustomGenderDropDownList("studentGender", "--Select--")
</td>
</tr>
</table>
<hr />
<footer>
<p>© @DateTime.Now.Year - My ASP.NET Application</p>
</footer>
As you can observe above lines of code., I have created simple student form where I have added a custom dropdown control
"@Html.CustomGenderDropDownList("studentGender","--Select--")".
MVC does not have this type of helper method in its assembly. It is an extension method.
Now run the application and check the result.
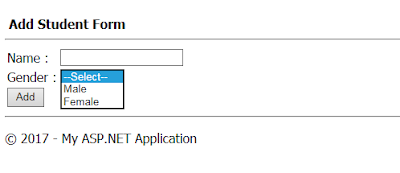
As you can see above picture, I am able to load gender dropdown items using custom dropdown helper method.
Follow below steps to implement custom dropdown helper method.
Step 1 : Create a class and name it as you like.
Step 2 : Add below line of code inside it.
using System;
using System.Collections.Generic;
using System.Web.Mvc;
using System.Web.Mvc.Html;
using System.Web.Routing;
namespace CustomHelperMethod
{
public static class HelperMethod
{
public static MvcHtmlString CustomGenderDropDownList(this HtmlHelper helper, string controlName,
string optionalLabel = "", object htmlAttributes = null)
{
IEnumerable<SelectListItem> selectList = null;
List<SelectListItem> genderList = new List<SelectListItem>();
genderList.Add(new SelectListItem() { Text = "Male", Value = "1" });
genderList.Add(new SelectListItem() { Text = "Female", Value = "2" });
selectList = (IEnumerable<SelectListItem>)new SelectList(genderList, "Value", "Text");
if (htmlAttributes != null && htmlAttributes is RouteValueDictionary)
{
RouteValueDictionary htmlAttr = htmlAttributes as RouteValueDictionary;
if (!string.IsNullOrEmpty(optionalLabel))
return helper.DropDownList(controlName, selectList, optionalLabel, htmlAttr);
else
return helper.DropDownList(controlName, selectList, htmlAttr);
}
if (!string.IsNullOrEmpty(optionalLabel))
return helper.DropDownList(controlName, selectList, optionalLabel, htmlAttributes);
else
return helper.DropDownList(controlName, selectList, htmlAttributes);
}
}
}
As you can have a look at above,
I have written a method called 'CustomGenderDropDownList' which is expecting multiple parameters such as name, optional label and html attributes.
Here ,
1. 'name' parameter indicates control's name
2. 'Optional Label' indicates whether or not you want to display '--select--' as first item in your dropdown control.
I added sample list of items and added them into IEnumerable of SelectListItem object
and pass them to 'helper.DropdownList' method and return it as . MvcHtmlString object.
Note: You can pull object list from DB and add them to 'IEnumerable of SelectListItem' object
That's it. You are done.
Note :
1. If you would like to add strongly typed dropdown control , write a helper method as below
public static MvcHtmlString CustomGenderDropDownListFor<TModel, TValue>(this HtmlHelper<TModel> helper, Expression<Func<TModel, TValue>> expression, string optionalLabel, object htmlAttributes = null)
{
--Add your logic--
}
Thank you.
Please feel free to ask your querries. I will be glad to help you all. And Hope this article will help you guys.
User reads below articles also:
No comments:
Post a Comment